Hibernate ORM doesn’t require the use of special build tools or IDE plugins. In particular, it does not depend on the use of a bytecode processor. But if you’re comfortable using an annotation processor, bytecode processor, or IDE plugin, we can offer some additional things which are otherwise impossible.
Tooling for Hibernate ORM
Tooling for Hibernate ORM includes:
-
Hibernate Processor, the annotation processor which produces the Jakarta Persistence static metamodel, validates queries at compilation time, and implements Jakarta Data repositories, and
-
the Gradle plugin and Maven plugin which perform optional bytecode enhancement at build time.
Relational schema management
Hibernate ORM comes with schema management built in. Hibernate is able to infer a schema from mapping annotations or XML mappings and automatically export the schema to the database, or verify that the inferred schema is consistent with the pre-existing schema. This functionality is especially useful for testing. The schema management tool can even truncate tables to clean up between tests.
For more advanced schema evolution needs, Hibernate can be used together with a tool like Flyway.
Tools for monitoring and profiling
Hibernate collects statistics as it goes. Your program can access this information via an API, but some environments report it automatically.
-
Hibernate Micrometer reports statistics via Micrometer. WildFly, Quarkus, and other Java containers have built-in support for Micrometer. You can learn more about using Micrometer in Quarkus.
-
Hibernate JFR reports persistence-related events to Java Flight Recorder. No configuration is required: just include the module
org.hibernate.orm:hibernate-jfr
as a runtime dependency, and you’re all set.
Hibernate Tools
Hibernate Tools is a suite of Eclipse plugins and part of JBoss Tools.
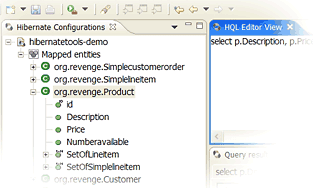
Console
The Hibernate Console perspective allows you to configure database connections, provides visualization of classes and their relationships, and allows you to execute HQL queries interactively against your database and browse the query results.
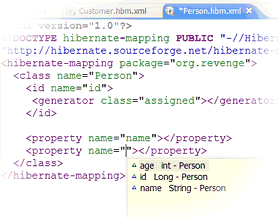
Mapping Editor
The Mapping Editor is an editor for Hibernate XML mapping files, supporting auto-completion and syntax highlighting. The editor even supports semantic auto-completion for class names, property/field names, table names and column names.
Reverse Engineering
Finally, the most powerful feature of Hibernate Tools is a database reverse engineering tool that generates domain model classes and Hibernate mapping files directly from a given database schema.